Vue Js Get Input Value by Id:In Vue.js, you can retrieve the value of an input element by its ID using a combination of the ref
and $refs
properties.
Firstly, add the ref
attribute to the input element and give it an ID value. Then, in your Vue.js component, create a reference to the input element using the $refs
property and the ID value of the input element. Finally, you can access the value of the input element by using the .value
property of the reference.
How can you Vue Js retrieve value of input element by ID?
This Vue js code allows you to get the value of an input field by its reference (ref
) using a button click event.
First, you create a Vue instance and define its data
object to include a result
property initialized as an empty string. Then, you define a method getValue
which will be called when the button is clicked.
Inside getValue
, you set the result
property to the value of the input field by accessing the $refs
object (which is a dictionary of references to the components in the template) and referencing the input field by the given ref
name (in this case, myInput
) and accessing its value
property.
Vue Js Get Input Value by Id Example
<div id="app">
<input type="text" ref="myInput" />
<button @click="getValue()">Get Value</button>
<p v-if="result">{{result}}</p>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
result: ''
}
},
methods: {
getValue() {
this.result = this.$refs.myInput.value;
}
}
});
</script>
Output of Vue Js Get Input Value by Id
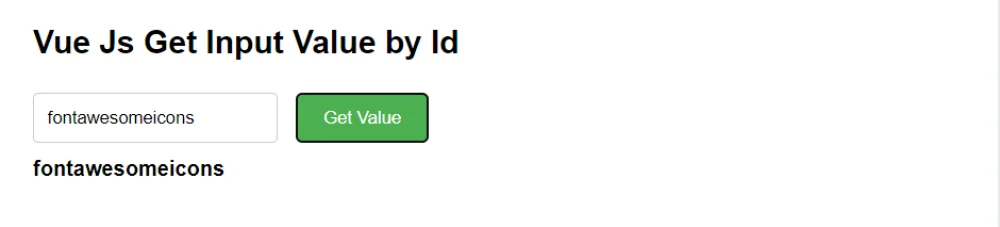